Laravel docker
A
re you familiar with Docker? If so, you may already know that today, most of the web applications out there are being dockerized. That’s means, using Docker, it’ll be much easier for the applications to run on different devices, no matter what operating systems or environments we’re working on. Because Docker ensures the consistencies of your dependencies in one place. It starts with the development from your local machine, then maybe some integration tests in the middle of it, and finally publish your app into the production environment.
Imagine you’re developing your app in the Mac Operation System right now, then you need the Swoole or other extensions for your PHP extension, which means you need to install those extensions manually in your local. Then, you may deploy your app to the Ubuntu server. What do you do? Of course, you need to install those extensions on the server, right? With different types of installation commands, you could encounter some errors, bugs, and so on. Then your colleagues may have a different operating system that required them to install those extensions in their local. So here, Docker comes to help. All of your application dependencies will be included inside the Docker. No matter what environment/OS you’re working on right now, everybody can run your app anywhere.
“Docker is an open-source engine that automates the deployment of any application as a lightweight, portable, self-sufficient container that will run virtually anywhere.” https://www.docker.com
In this article, I’ll show you the easiest way to run your Laravel application using Docker. For the brief, first, we’ll create a custom image using Dockerfile. Then later, we’ll create a docker-compose to make things even easier.
Content Overview
- Dockerfile for Laravel
- Running the Image with Docker Compose
- MySQL DB & Artisan Queue Services
#1 Dockerfile for Laravel
Dockerize your Laravel app isn’t very difficult if you’re already familiar with the basic syntax of Docker. I assume you have a Laravel project in your local, if you don’t, you can create one with this command.
composer create-project laravel/laravel --prefer-dist my_app
Then, cd
(change directory) to your project, and create a new file named Dockerfile. Notice that the name of the file starts with capital D without any extensions.
FROM php:7.4-fpm-alpine | |
RUN docker-php-ext-install pdo pdo_mysql sockets | |
RUN curl -sS https://getcomposer.org/installer | php -- \ | |
--install-dir=/usr/local/bin --filename=composer | |
COPY --from=composer:latest /usr/bin/composer /usr/bin/composer | |
WORKDIR /app | |
COPY . . | |
RUN composer install |
Dockerfile
On line 1, we’ll use PHP version 7 as our base image. Of course, feel free to change the PHP version to whatever you want. Then on lines 3–7, that’s where you can install the dependencies that may be required in your project. Right now, we just need the composer for installing Laravel dependencies for our app. On lines 9–11, we change the directory into our app, then copy all the files from our local machine into the /app
of our Docker container, then run composer install
.
That’s it. We’ve created a custom image for our app. It’s time to run the image. You can run with or without docker-compose. For running our app without the docker-compose, here are the steps we can do.
First, open the terminal and type this to build your Dockerfile.
docker build -t my_app .
Then, we may need to see the Image ID using this command.
docker image ls
Copy the image ID from the lists, and then we can run the image.
docker run -p 8000:8000 -d <imageID>
The flag -p
above is exposing our main machine port, into the docker port. Then for the -d
flag means that we’ll run the image in the background.
After the image is successfully running, we can run the server by executing the php artisan serve
command inside the running container. First, we need to know what is the name (or container ID) of our app, by using this command docker ps
. Then, we can get access to its command line using this command:
docker exec -it <container ID> sh
Inside the container command line, we can start the server using the usual php artisan serve
.
php artisan serve --host=0.0.0.0
From your local machine, open localhost:8000
and you’ll see that our Laravel App is successfully running from the docker container.
#2 Running the Image with Docker Compose
Okay, that’s a lot of commands to run an image from the Docker. Fortunately, docker-compose will make things easier. Let’s create a new file named docker-compose.yaml
inside our root project.
version: '3.8' | |
services: | |
main: | |
build: | |
context: . | |
dockerfile: Dockerfile | |
command: 'php artisan serve --host=0.0.0.0' | |
volumes: | |
- .:/app | |
ports: | |
- 8000:8000 |
docker-compose.yaml
What the commands above do is pretty similar to what we did manually before. For running the app, we can easily type this command:
docker-compose up
Voila, our app is running from the Docker container using the docker-compose.
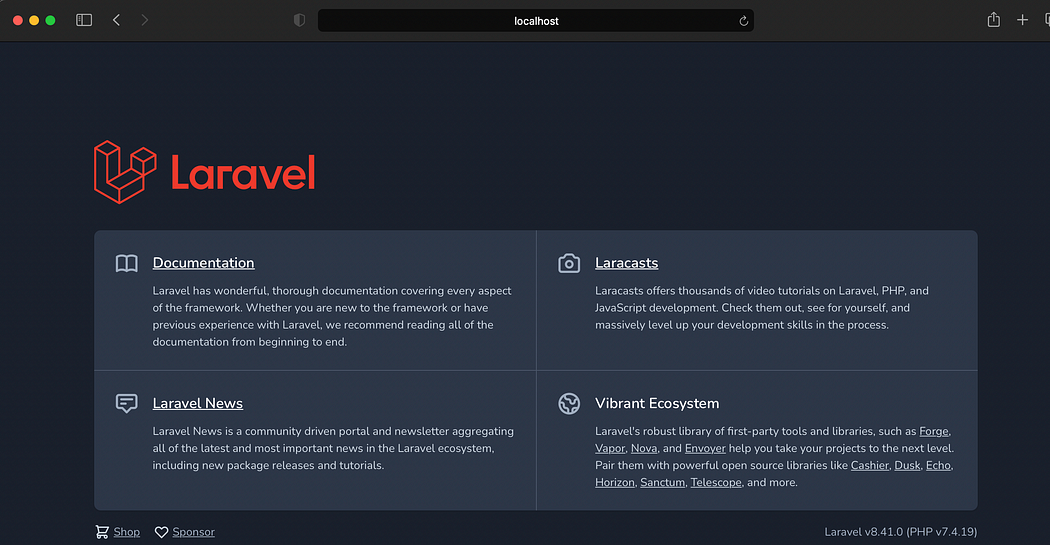
Comments
Post a Comment